ES6 cheatsheet — Helpful array functions
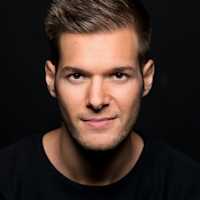
Serban Mihai / 20 October 2018
~1 min read
**from**
const inventory = [
{name: 'mars', quantity: 2},
{name: 'snickers', quantity: 3}
];
console.log(Array.from(inventory, item => item.quantity + 2)); // [4, 5]
**of**
Array.of("Twinkle", "Little", "Star"); // returns ["Twinkle", "Little", "Star"]
**find**
const inventory = [
{name: 'mars', quantity: 2},
{name: 'snickers', quantity: 3}
];
console.log(inventory.find(item => item.name === 'mars')); // {name: 'mars', quantity: 2}
**findIndex**
const inventory = [
{name: 'mars', quantity: 2},
{name: 'snickers', quantity: 3}
];
console.log(inventory.findIndex(item => item.name === 'mars')); // 0
**fill**
method takes up to three arguments value, start and end. The start and end arguments are optional with default values of 0 and the length of the this object.
[1, 2, 3].fill(1); // [1, 1, 1]
[1, 2, 3].fill(4, 1, 2); // [1, 4, 3]
You can find a more complete ES6 cheetsheet on my Github page.
P.S. If you ❤️ this, make sure to follow me on Twitter, and share this with your friends 😀🙏🏻