ES6 cheatsheet — Async Await
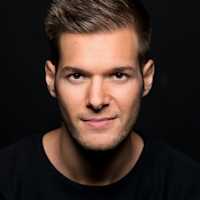
Serban Mihai / 20 October 2018
~1 min read
- Async/await is a new way to write asynchronous code. Previous options for asynchronous code are callbacks and promises.
- Async/await is built on top of promises. It cannot be used with plain callbacks or node callbacks.
- Async/await makes asynchronous code look and behave a little more like synchronous code.
Note that await
may only be used in functions marked with the async
keyword. It works similarly to generators, suspending execution in your context until the promise settles. If the awaited expression isn’t a promise, its casted into a promise.
async await
allows us to perform the same thing we accomplished using Generators and Promises with less effort:
var request = require('request');
function getJSON(url) {
return new Promise(function(resolve, reject) {
request(url, function(error, response, body) {
resolve(body);
});
});
}
async function main() {
var data = await getJSON();
console.log(data);
}
main();
Under the hood, it performs similarly to Generators.
You can find a more complete ES6 cheetsheet on my Github page.
P.S. If you ❤️ this, make sure to follow me on Twitter, and share this with your friends 😀🙏🏻